In-Depth
MonoDroid Tutorial Part 2: Building a MonoDroid App
In the second part of this tutorial series, Wallace McClure walks you through the basics of creating a MonoDroid app.
- By Wallace McClure
- February 1, 2011
Update: The Mono Project on April 6 released Mono for Android 1.0, including the updated Visual Studio plugin.
Download the tooling and files here.
In the first part of this MonoDroid tutorial, we looked at the basics of Android and MonoDroid. Now let's look at the initial sample MonoDroid app. Here's a series of steps.
Once the MonoDroid plug-in is installed, let's create a project. Figure 1 shows the different project types you can create with MonoDroid. With this example, we'll examine the default MonoDroid app. The default project has a button whose test is updated with the number of times that the button has been clicked.
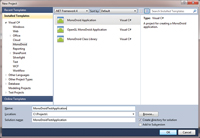 [Click on image for larger view.] |
Figure 1. Available project types with MonoDroid. |
The Visual Studio Solution Explorer in Figure 2 shows the resulting project. There are several items of interest in the project that need to be pointed out. First, Activity1.cs is the class that's created that contains most of the resulting executable logic. Second, the Resources directory has a series of items that will be embedded into an application. These resources include the UI and images and will be marked as an AndroidResource for their Build Type. Finally, the starter UI is contained within the file main.xml.
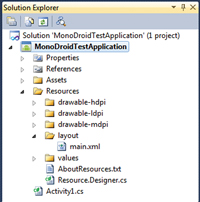 [Click on image for larger view.] |
Figure 2. A typical MonoDroid project shown in the Visual Studio Solution Explorer. |
Designing an Android UI
Most developers are familiar with the Visual Studio design surface for building Windows Forms and WebForms applications. Building a UI for Android will be nearly a foreign concept for these developers. An Android UI will have no design surface support in the initial release of MonoDroid. However, have no fear. Because there hasn't been a lot of design surface support in the Android SDK until recently, there are some third-party developers who've built standalone tools for building an Android UI. I've been using DroidDraw, and there are others.
This is an example definition for an Android UI:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<Button
android:id="@+id/myButton"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
</LinearLayout>
Android contains many of the UI widgets that developers and users are expecting. These UI widgets include text boxes, labels, drop-down list boxes, repeating elements, images and other types of controls. This example contains a button, and the button will be updated after each click.
The Code
The code for the sample application is shown in Listing 1. A few of the items to pay attention to are:
Android Namespaces: There are a series of Android-specific namespaces that provide a .NET Framework layer your application can call into. Remember, MonoDroid isn't about Windows Forms or Windows Presentation Foundation (WPF) running on Android. You're building a native Android application with C#/.NET. You still need to learn the specifics of Android.
Activity Attribute: There's an Activity attribute that contains information that will be displayed when your app is installed into a device or the simulator. You won't see an AndroidManifest.xml in a project by default. If you do decide to, you can manually create it or create it in the project properties. This file sets the permissions that an application needs to run. This can include things like the version of the API required for an application to run as well as access to what features on the device an application needs. These can be set by editing the file by hand or by modifying the application's properties. The properties look something like Figure 3.
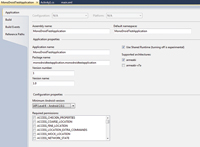 [Click on image for larger view.] |
Figure 3. Setting application permissions. |
The AndroidManifest.xml file is stored in the Properties folder of the project in Solution Explorer. The contents of the AndroidMani-fest.xml are shown here:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android=
"http://schemas.android.com/apk/res/android" package=
"monodroidtestapplication.
monodroidtestapplication"
android:versionCode="1"
android:versionName="1.0">
<application android:label=
"MonoDroidTestApplication">
</application>
<uses-sdk android:minSdkVersion="6" />
<uses-permission android:name=
"android.permission.
ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.BATTERY_STATS" />
</manifest>
Contained in this version of the AndroidManifest.xml file is some information concerning the application version, a name for the label name for the application, and the minimum version of the API that's required. In this case, SDK 6 means Android 2.01.
Also included are the features that the application will need access to on the device. In this case, the app is requesting access to the coarse location information, fine location information and battery status. Note that fine location information implies coarse location information. This data will be compiled into your application. If your application is deployed to the Android Marketplace, this information will be presented to the user regarding device resources that the application needs.
Your class will inherit from an Activity and there are a series of events you can override. The example app merely overrides the OnCreate event. Also, the OnCreate event loads the application UI by calling SetContentView(Resource.layout.main) and passing the reference to the main layout.
The final step in this is to create a reference to the button that's in the main.xml UI. Once the reference is created, a .Click event is created programmatically. When the user clicks on the button, the event will change the text in the button, as shown in Listing 1.
When looking at the code, you're probably wondering where the references to Resource.id.control and Resource.layout.main are stored. There's a file in the Resources folder named Resource.Designer.cs that contains this information. It's auto-generated, so you don't typically want to touch it. The file will look something like Listing 2 for our sample project.
This file provides your application with the references necessary to relate controls in the UI to your code. Additionally, it will help in providing IntelliSense support within Visual Studio. Look for more additions and changes in this feature after the 1.0 release.
Compiling and Running
Now we've reached the exciting part. You've fixed all the bugs in your code and it compiles. When you start a debugging session, you'll get a dialog like the one shown in Figure 4. This dialog is asking you to select a device to deploy the application to. These two devices listed are for:
- HT06LHL08688. This is a physical device attached to my development system. Specifically, this is my HTC EVO 4G running Android 2.2.
- Emulator-5554. This is an emulator session on my local system that I set up. If there are other emulator sessions running, they'll appear listed here as well.
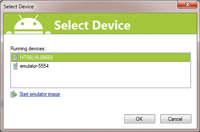 [Click on image for larger view.] |
Figure 4. Deploy your application to either an emulator session or an attached physical device. |
After this, there are some messages regarding the status of deploying the Mono runtime and copy-ing the application to a device. The app is stored in the emulator with the other applications, as shown in Figure 5. It's interesting that the name displayed for the user is the same as the value for the Label property in the Activity attribute.
Once these steps are completed, if you have a new instance of the simulator running, you'll need to open the simulator and your application will be running.
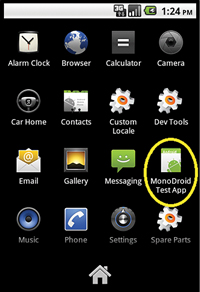 [Click on image for larger view.] |
Figure 5. The MonoDroid Test App appears in the app screen. |
Congratulations! Your first Android application built with MonoDroid is up and working.
Great, but What About…
There are a couple of other items that come up in a discussion of MonoDroid. First is the issue of pricing. While no formal pricing has been announced at this point, MonoDroid will most likely be packaged and priced similarly to MonoTouch. MonoTouch has the following versions:
- Trial: The trial version doesn't cost anything, but it doesn't have the ability to deploy an application to a physical device.
- Single Developer: The single developer version has a list price of $400. It's designed for a single developer or small company building applications.
- Enterprise: The Enterprise version is licensed to a company and has a list price of $800 per developer. Volume discounts are available.
In April 2010, Apple created a stink in the development world by changing the licensing for its iPhone (iOS) SDK. This licensing change created fear, uncertainty and doubt regarding the use of non-Apple tools. This was in spite of the fact that Apple never removed any applications from the AppStore not written in its tools and language. In September 2010, Apple changed its position and relaxed its official stance on non-Apple tools. Unfortunately, this issue still causes some concern in the minds of many developers.
In August 2010, Tom Hanrahan of the Microsoft Open Source Technology Center stated that Mono would fall under the Microsoft Community Promise Agreement. This was in reference to questions about the Oracle versus Google lawsuit regarding the use of Java in Android. It's assumed that this means Microsoft won't sue over MonoDroid's use of the defined set of .NET APIs. Given Google's effort to attract more developers, it's doubtful that Google will somehow attempt to disallow from the Android platform applications written in MonoDroid.
Another open question is support for Silverlight. There's been a lot of talk recently about HTML5, Silverlight and where these products fit into the marketplace. Novell has a cross-platform implementation of Silverlight called Moonlight.
One question I've fielded recently is, "What's the status of Silverlight on Android?" The thinking seems to be that, if MonoDroid is .NET for Android, and Silverlight is a UI for .NET, then Silverlight should be available for Android. I've spoken about this subject with Joseph Hill, the program manager for MonoDroid at Novell. He states that the company is looking at adding Silverlight/Moonlight support to MonoDroid in the future, but it's not in its plans for MonoDroid 1.0.
We've seen the growth rates of Android. We've seen the interest that .NET developers have in developing on Android, and we've also seen that .NET developers can target Android devices. I'm excited about the future of mobile development on Android thanks to MonoDroid.